Chessy - Episode 1
“I want to create an application to reproduce chess matches” is what I said in the previous post, and here I publish the first notes about what I arbitrarily decided to name chessy.
In this post, I start mentioning how chess games are recorded, then I talk about the process I follow to work in this project, I then describe the first two components I worked on (the UI and its data) and, finally, conclude with some implementation details.
I assume the reader is familiar to what “chess” is and how to play it (roughly, at least). But in case you’re not that reader and you feel a bit lost like me watching cricket, here’s my good deed: Chess is played by two players, with Black and White pieces, on an 8x8 board, in turns, and in each turn something changes on the board: pieces are moved, taken or transformed. You’re welcome (?).
The interesting part (for me) is that chess games are recorder in text format, by simply writing down all the movements that have ocurred (besides other information like date, place, player names, etc). The standard format to record a game is PGN and you can look at examples in its wikipedia article. I found that tournament’s websites, or even the federation, publish the games using this particular format. For example: Norway Chess, or FIDE World Championship 2018. These are the type of games I aim to reproduce.
Process
There’s a principle I once read called Tracer Bullet 1 that suggests that one should first create an end-to-end skeleton -very simple and perhaps overly mocked- of the whole system in order to use it as a ‘trace’ for the following ‘bullet’ - the complete system. This differs from “prototyping” where work done at early stage is thrown away. Although I have an idea of the system I’m building, I don’t have full clarity about the UI, the specific format and data I need to process and handle, or the communication among components. I therefore create simple (though working and tested) components, that can be refactored and fleshed out in future iterations.
The first components I begin with are the user interface (UI) and the data driving it (UI Data).
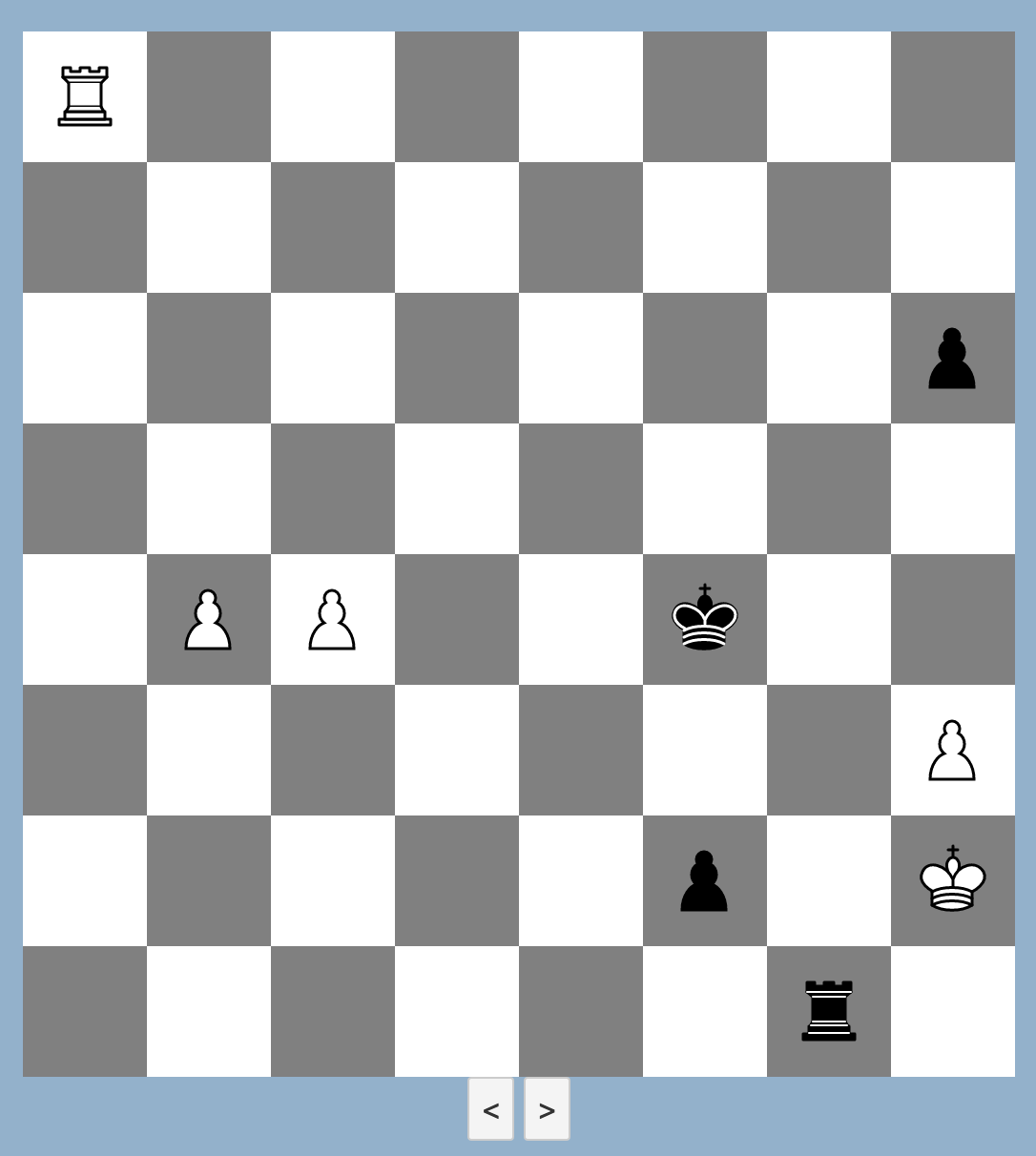
The UI
The UI consists on a chess board, the pieces on top, and two buttons -previous and next- so the user can move the game back and forth.
I want the UI should to be extremely simple, limited to display the game at any point on time - no more. I don’t want the UI to get involved in “business logic” (in this case: calculate the positions of the pieces on the board, or detect things like a check) for two reasons: I plan to build more than one app - so “the simpler the better” - and because that logic can be tricky - better to have only one place to maintain.
The UI Data
To support such a simplistic UI, its data must be ready to use. In the reproduction of chess matches it means that the data must be an ordered list of board positions. Since the games I use (PGN Format) are basically a list of movements instead, these should be pre-processed to my convenience: from list of movements to list of board positions.
Those board positions will be written in FEN Format (luckily there’s a standard for that as well) which consist in a string with letters, numbers and slashes. For example, a FEN board position like rnbqkbnr/pppppppp/8/8/8/8/PPPPPPPP/RNBQKBNR
2 means: Starting from the top-left square, there is a rook (r), a knight (n), a bishop (b), a queen (q), a king (k), a bishop, a knight, and a rook; in that order. In the next row (indicated by the slash /
) there are only pawns (eight p’s). In the following row there are 8 empty squares (indicated by the number), and so on. Lowercase letters represent Black pieces, uppercase letters represent White pieces.
Implementation Details
My first UI is web because is the quickest for me to iterate with. I made it using Svelte because this framework intrigued me for a while: it adheres to the reactive and component-based philosophy, focuses strongly on performance, and has a cool logo (?). I programmed in TypeScript where I could 😎, and JavaScript everywhere else 3 😢.
The webapp plays the game indicated by the gameId
query parameter (the ?gameId=1
in the URL), and can be 1,2, or 3. Of course, in another iteration I will add more matches to be selected from a list or menu.
The first version is hosted in vercel (amazing product this people have!) and the source code can be found in github. Check it out!, and try the 3 games by changing the last number in the URL (the games are from the 2013 World Championship, between Anand and Carlsen).
The UI Data (list of FEN positions) is stored as JSON files. Why JSON? because it’s very popular and every language/framework has libraries to parse them. Plus, it’s simple to extend it with more information (later). I store them as files in S3 just for simplicity - I will surely switch to a cloud-based database later. You can see an example of my UI Data by opening one game.
And that’s all for today!
You might be wondering “how did you generate that list of board positions from the PGN file?”. Thanks for asking! That’s a perfect topic for the following post!◆
-
I read about it in The Pragmatic Programmer book. The proper principle is a bit more specific than my free-style interpretation of it. ↩
-
I am intentionally skipping the last part of FEN string, which indicates who’s turn is, whether castling is still valid movement, and more. ↩
-
Svelte introduces some fancy syntax on
.svelte
files (like$:
) and I haven’t managed to add Typescript support there… yet. ↩